Actions
The functionality of Standard Services
is available to get information about application services. Maximo Asset Management has multiple application services - aka Appservices, which provides service methods that act on MBOs to perform a business task. While most of these calls end up modifying the state of the system, some of these calls are just to get information. You can use the JSR 181
annotations to expose these methods as WebMethods
, which can be accessed using SOAP and REST calls. For the purpose of this discussion, we are only going to focus on the RESTFul aspects of these methods.
Simply put, you can add a WebMethod
to any existing Maximo application service by extending the service class and adding a method. This example is from extending the Workorder
Service (psdi.app.workorder.WOService
):
@WebMethodpublic void approve(@WSMboKey(value="WORKORDER") MboRemote wo, String memo){wo.changeStatus("APPR",MXServer.getMXServer().getDate(),memo);}
Next you want to make sure that your method is available for REST API calls. You can select any work order record and use the rest API to get the set of allowed actions on that resource as shown in the following query:
GET /oslc/os/mxapiwo?oslc.where=wonum="1001"&oslc.select=allowedactions
You will see the list of allowed actions, which are WebMethods for the service corresponding to the root object of the object structure along with the list of actions registered with the object structure (using the application menu “Action Definition”). The resulting JSON provides the JSON schema for web method as well as the HTTP method and the name of the action, which can be used to invoke the action. The following sample call demonstrates how these methods are invoked:
POST <uri of the workorder 1001>?action=wsmethod:approvex-method-override: PATCH
Post body:
{"memo":"Testing"}
Note you need to set the request header x-method-override
as PATCH
for invoking this action API, as this is operating on an existing MBO (the first parameter to the method). This MBO reference is derived from the request URI (the URI of the work order 1001) and passed into the method as part of the API invocation. The JSON schema for the payload reflects the parameter data types (in the method) and the parameter names.
The query parameter action=wsmethod:approve
is the fully qualified name of the action. The format is <action type>:<name>
where name
is the java method name for the action type wsmethod
.
In additon to this, we also support the concept of streaming action apis where one can directly access the REST request input stream. In this model you can define a java method with one and only one method parameter of type com.ibm.tivoli.maximo.oslc.provider.OslcRequest
. An example is shown below
@WebMethodpublic void loadFile(OslcRequest request){//you can now use the request to get the query params as well as the inputstream likeString params1 = request.getQueryParam("param1");InputStream is = request.getInputStream();//use the input stream to read the blob which is in the body of the request....}
However there are limitations with overloaded methods, where overloaded methods are not supported for REST API calls.
There are methods available in the out of the box service and you can use allowedactions
to see these methods.
In addition to webmethod
based actions, the REST APIs also support scripted actions. You can write a REST action code by using automation scripts. A simple example below will explain this concept.
Firstly, you create an automation script in the Automation script application by using the Create > Script For Integration option. You select the object structure, then select the type of script as Action Processing
, and give a name for the action. You can write a script (in any language - python
, js
) by using the implicit variable mbo
. For example, the following script changes the status of Asset to OPERATING
:
mbo.changeStatus("OPERATING",False,False,False,False)
Save the script. Note that you did not write any code to commit the modification to the Asset MBO. The REST API framework commits the transaction after the method completes.
Next you register that script with the object structure in the Object Structure application. Select the Action Definition action and set the script as an action to the object structure. Name the action same as the name of the script and click OK to save the configuration.
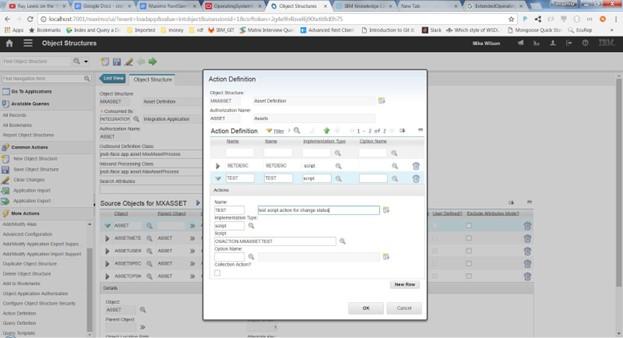
Next you can invoke this script similar to the following request:
POST <uri of the asset 1001>?action=TESTx-method-override: PATCH
The asset status changes to OPERATING
. Note that if you want to get the changed Asset in response, you can add the request header properties
with your desired value.
You can also use this actions rest APIs to invoke workflows
. The following example shows how the ABC
workflow for the asset is invoked:
POST <uri of the asset 1001>?action=workflow:ABCx-method-override: PATCH
For non-interactive workflows, this is all you need to do to initiate it. Interactive workflows require user interaction by the placement of assignments, input, and interaction nodes. Interactive workflows will be covered in the “Interfacing with the Workflow Engine” section.