Attribute launch point
Attribute launch point
You can use the Attribute launch point to customize the field validation, action, initialization, and lookups in the scripting framework. The script can access to the event MBO using the implicit variable “mbo” as well as all the related MBOs. Additionally,the modified attribute would also be available implicitly as a variable inside the script. The variable name would be the lower case value of the modified attribute name. The script will have access to the event MBO using the implicit variable “mbo”, the MboSet as well as all the related MBOs. The following examples explain the Attribute launch point. To design scripts using Attribute launch point, start the Create Scripts with Attribute Launch Point wizard.
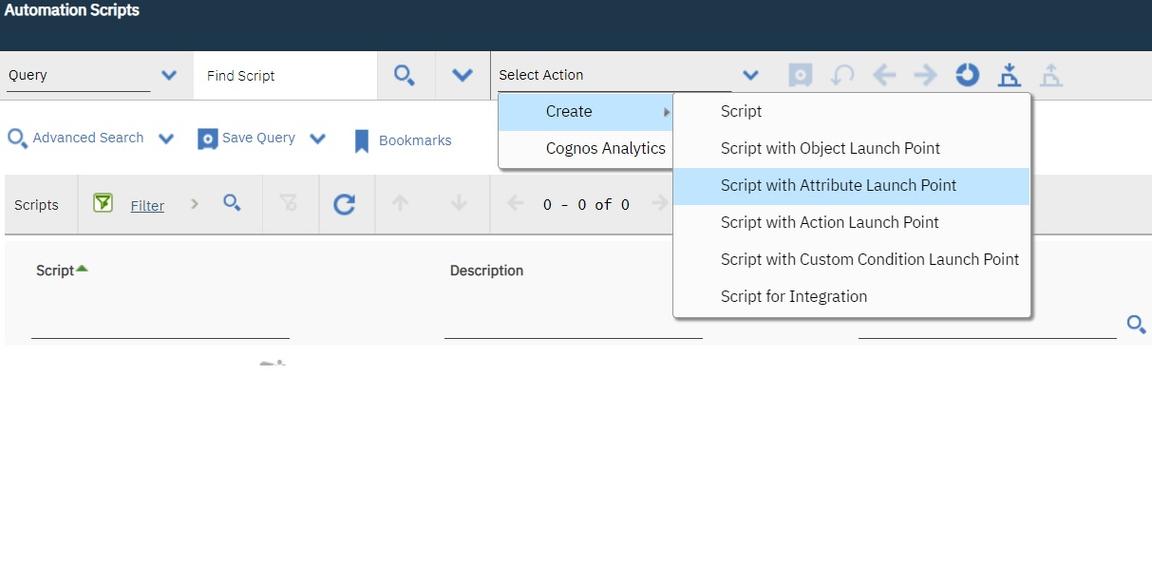
Validate and action events
You can customise the Asset MBO by adding custom business logic based on the Asset purchase price or attribute purchaseprice in Asset value. For example, to set the vendor field to required or not required based on the purchaseprice attribute value and also to calculate the replacement cost based on the purchase price. You should also ensure that the purchase price does not exceed a maximum allowed value.
The logic has to be injected at the modification of the purchasprice attribute value, which implies that it should be modeled as an Attribute Launch Point. There is a validation element in the requirement, where the purchaseprice cannot exceed a certain limit. There is also an action element wherein the state of the vendor attribute and the replacementcost value gets set based on the purchaseprice value. The first requirement (of validation) should be handled by the Attribute validate event and the second requirement should be handled in the Attribute action event. The following figure shows the events available in Atribute Launchpoint wizard.
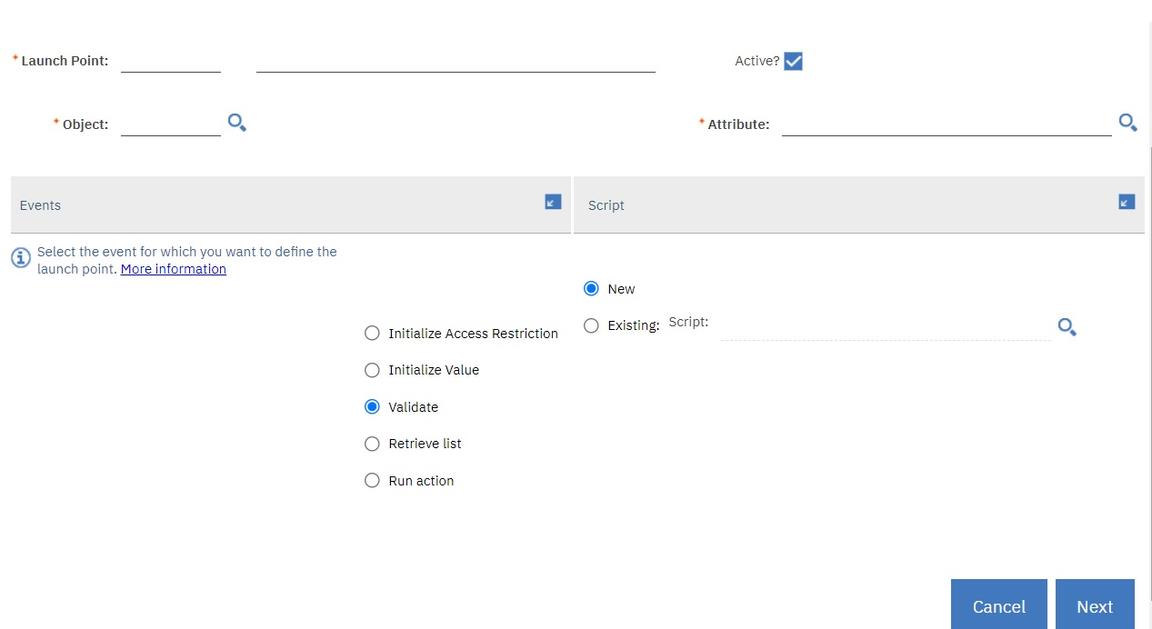
The “validate” script should be quite simple:
if purchaseprice > 200:service.error("some","error")
You can use the implicit variable purchaseprice
, which is attribute on which the launch point is based on, and also use the `service.error(..) to throw the needed MXException for this validation.
The following script would be for the Attribute Action event:
if purchaseprice >= 100:vend_required=Trueelse:vend_required=Falserc = purchaseprice/2
This script leverages two explicitly defined OUT variables, vend
for attribute “vendor” and rc
for attribute “replacementcost”.
By leveraging the implicit variable vend_required you can control the state of the “vendor” attribute to required or not required. You can also set the “replacementcost” by setting the rc
.
Another way to do this without using any of these explicitly defined variables is to leverage the standard MBO APIs and the implict “mbo” variable.
from psdi.mbo import MboConstantsif purchaseprice >= 100:mbo.setFieldFlag("vendor",MboConstants.READONLY, True)else:mbo.setFieldFlag("vendor",MboConstants.READONLY, False)mbo.setValue("replacementcost",purchaseprice/2)
If you use the standard MBO APIs and the implicit “mbo” variable, you have more control but you must be aware of the MBO APIs.
For example, if the “replacementcost” field is readonly, both scripts will fail as the MBO framework will reject the setValue
call
on that attribute.
A possible workaround is to leverage the variable binding metadata - “Suppress Access Control”. By setting this metadata, the scripting framework can set the rc
variable value to the “replacementcost” whether it is readonly or not.
However, this will only work if the script code uses the variable binding. For scripts that use the MBO APIs, the script code will need to leverage the MboConstants setting to achieve the same.
from psdi.mbo import MboConstants..mbo.setValue("replacementcost",purchaseprice/2, MboConstants.NOACCESSCHECK)
Another use case is to make the calculated field created for the Asset sparepart total quantity calculation more real-time. For example, if You change the quantities of the related spareparts you should see the calculated field [sparepartqty] value change real-time. To achieve this, you need to create a script - attribute launchpoint for attribute “quantity” for “sparepart” object with the “Action” event. The explicit variable binding is shown in the following table:
Variable name | Variable type | Binding |
---|---|---|
sptqt | INOUT | &owner&.sparepartqty |
The variable sptqt is bound to the owner’s [Asset mbo] sparepartqty attribute. You must set the sptqt variable with the “No Access Check” as it is marked as readonly by our original [Object launch point] script when initializing the Asset MBO. The variable quantity, which is the attribute on which the script is listening to would be implicitly injected in the script as that is the attribute on which the script is listening. The following script shows how it will look:
sptqt=sptqt+quantity-quantity_previous
The use of the implicit variable quantity_previous represents the value of the quantity attribute before the modification, which is the value at the initialization of the sparepart MBO. The variable quantity holds the current modified value of the quantity attribute.
The script and the Object Launch point script can give you a complete calculated field logic implemented using the scripting framework with three lines of script and simply executing the Script Wizard.
Note: Maximo formulas provide a simpler way to handle this use case.
Creating lookups using scripts
You can create lookups using the attribute launch point Retrieve List option. As with any
attribute launchpoint, you must hook the script up with the MBO attribute on which you
want the lookup to happen. While writing the script is enough for lookups to work from REST APIs (GET getlist~attr
), it is not enough for lookups to work either in Graphite or in classic UI.
For classic UI you are required to make an entry in the lookups.xml
for the lookup dialog. You also need to associate the
lookup name to the MBO attribute in the corresponding presentation xml(s). In the following example, you
write a lookup for the “address5” attribute in the Address MBO for the multisite application. The
“address5” maps to a country name using the country code. For example, you want to use some of the
external rest APIs available that provides the list of countries. There are a number of APIs that can be used. However, for this example, a simple HTTP GET call with a user/password (basic auth) and a response is being used:
{"USA" : "United State Of America", "CAN":"Canada", "GBR": "United Kingdom"}
You must then write the “retrieve list” script. This example is in Nashorn JS, as a sample JS and to leverage some of the native json parsing capabilities of Nashorn JS.
importPackage(java.util)importPackage(Packages.psdi.server)var jsonResp = service.httpget("..url..","..userid..","..password..");var countries = JSON.parse(jsonResp);var countriesSet = MXServer.getMXServer().getMboSet("COUNTRY", userInfo);for(var cnt in countries){var cntMbo = countriesSet.add();
The implicit variable listMboSet
holds the MBOSET to be
used in the lookup. The target MBO attribute where the value is going to be set has a different name
(address5) from the src attribute name (“name” in COUNTRY MBO). Therefore, you must use the implicit vars
srcKeys
and targetKeys
to let the system know where to set the selected value.
Init and init value attribute events
The Init and Init Value attributes events help set the attribute flags (init event) and initialize the value (init value event). You can also do this using the Object init event but that can affect performance. These Attribute events leverage the Maximo frameworks for just-in-time initialization. The scripts are called only if the framework needs to access that attribute. Object init event would initialize the attribute whether it is needed or not.
The following sample script is used to initialize the “priority” attribute:
if mbo.toBeAdded()==True and mbo.getString("site")=="BEDFORD":thisvalue = 1
Note: The implicit variable thisvalue
represents the attribute for this launch point.
Similarly, the “init” event provides implicit variables for setting the required/readonly and hidden flags.
Variable name | Variable type | Purpose |
---|---|---|
thisvalue_required | OUT | Sets the attribute to be required or not |
thisvalue_readonly | OUT | Sets the attribute to be readonly or not |
thisvalue_hidden | OUT | Sets the attribute to be hidden or not |
The following sample script is for this event:
if mbo.toBeAdded()==True and mbo.getString("site")=="BEDFORD":thisvalue_required = True
You can set flags to the attribute (for this launch point) conditionally and the framework will invoke this only when it is being referred to.
Setting Maximo lookups
Support for getList was already there – Retrieve List event for Attribute Launch Point. We had no way to set the lookup name. This feature introduces how we can conditionally set the lookup name using scripting – in the Attribute init value event. This will get leveraged in the retrieve list event when the lookup is clicked by the end user.
Note that the lookupname
is the implicit variable where the script will set the lookup name - from the lookup xml library.
The below code just sets 2 known lookup names - just as a sample to showcase how we can set the lookups dynamically.
if mbo.isNull("assettype"):lookupname="numericdomain"else:lookupname="alndomain"
Note that this needs to get backed up by the script at the Retrieve List launchpoint. A sample is shown below:
if mbo.isNull("assettype"):domainid="ASSETP"else:domainid="ASSETP2"